Over the last few years, Slack has skyrocketed to become one of the most widely-used business platforms around. The company’s commitment to creating minimalist, yet useful software has won over business people worldwide. Slack has offered a number of ways to get more out of their platform beyond just vanilla Slack. Slack allows for endless integrations through use of their API, which is one of the best around.
One of the most useful applications of this API is the creation of Slack Bots. Slack defines their bot applications as “virtual team members” that can help you manage tasks, among other things. In this tutorial we will:
- Learn about the Slack API.
- Learn about Slack Bots.
- Create your own Slack Bot that generates random, useless facts.
Here’s an example of the end result:
So let’s go ahead and begin!
Slack API
If you’ve read this far, I’m assuming you’re already familiar with Slack as a platform. If not, it’s simply a tool that helps teams communicate – sort of like Discord, but more professional. With so many businesses using Slack, the need for a comprehensive API became apparent, and Slack has certainly delivered.
Slack’s platform has a number of APIs that allow for the creation of “apps.” These APIs are:
You can read more about each of these more in-depth, but this tutorial will be using the Events API and the Web API.
The Events API – Web API Relationship
Creation of complete Slack apps generally requires use of the Events API and the Web API. This is because these two APIs do very similar things, but with different end results.
Slack likens these two APIs to players in a game of ping-pong. Whenever an “event” happens within a slack channel, it pings information about this event over to your app. This is what the Events API does. Whenever your app processes the received information and wants to make a change within a slack channel, it pongs commands back to Slack. This is what the Web API does. These APIs are both notifiers and observers, just functioning in two different directions.
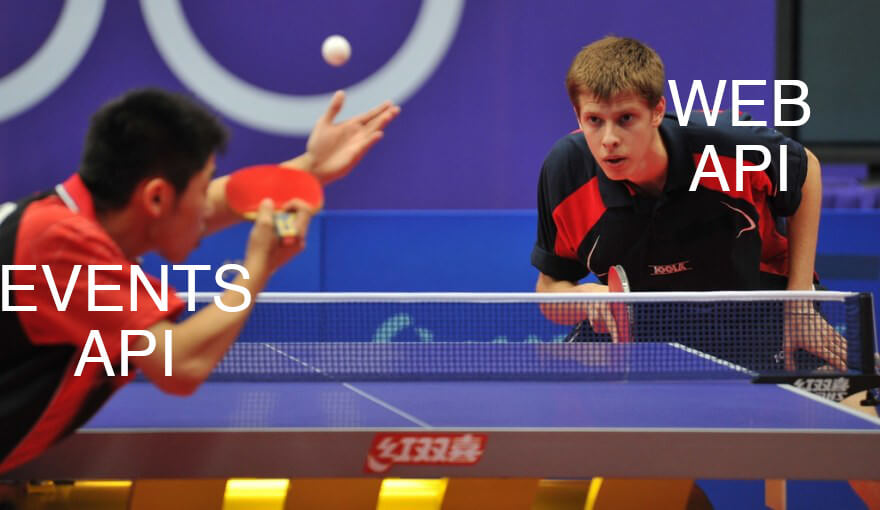
Your app will just be a fancy game of ping-pong.
Slack Bots
There are a number of different kinds of apps you can make using the Slack API. Slack apps can manage workflows, interpret and act upon user messages, connect with other APIs.
Bots are one such app that can make your channel more useful. Slack Bots listen for commands from users and spit back relevant information. For example, you could create a bot that responds with the current user’s local temperature when they type “/temperature” or the company’s sales for the current quarter by typing “/revenue.” You can integrate pretty much any information you could pull from an API into a Slack Bot command.
In this example, we are going to make a Slack Bot that listens for the “/todo” command and returns the current user’s list of pending tasks to do. We will be using the Tallyfy API to do this as Tallyfy is a business process management tool that manages tasks.
Creating our Slack Bot
Required Knowledge:
- php
- cURL
Step 1: Create the App
The first step to creating our Slack Bot is to simply create an app within Slack. To do so, click this link, name your app, and choose a workspace for the app to be in.
Then give the app a description, image, and background color.
Step 2: Create Request URL
Now that we’ve got our app made in Slack, we need a place to actually host our app on our end. This means we’re going to have to make a php file that listens for events from Slack’s Events API, processes these events, and sends commands back to Slack.
I’m naming the file “todoBot.php” and am hosting it on my own site, so my url will be .
Step 3: Setup “/todo” Command
Now we need to tell our app which commands to look out for. To do this, go to the tab labelled “Slash Commands” and click “Create New Command.” We’re now prompted with a form that will let us set up our command.
Make sure the “Request URL” field points to the place we are hosting our php file. Fill out the rest of the form similarly to how I have it below and click save.
Now everything is set up on Slack’s end and its time for us to code!
Step 4: Activate Incoming Webhooks
This next step will let our app point to where we want changes to be made in our Slack workspace. To do this, we need to go to the tab labeled “Incoming Webhooks,” and turn the “Off” switch to “On.”
We must then choose a channel we want our app to make changes to. Choose the channel you want to install our app to.
Now, if we scroll down, there will be a URL under “Webhook URL.” Save this URL as we will need it later.
Step 5: Make Request to Tallyfy API
In order to communicate our tasks to Slack, we have to make a request to our Tallyfy API. Note that while I am using Tallyfy’s API to get data, you could pull data from any API and pass this over to Slack (I’ll show an example of this at the end).
We will be using cURL in php to make our API request. Most APIs make use of cURL, so it is a valuable process to understand.
Within our conditional statement, we want to initialize our cURL, tell it where to pull data from, and set our HTTP headers so that the Tallyfy API authenticates us. We then execute and close the cURL. The request returns a JSON of our Tallyfy organization’s outstanding tasks!
//Initiate cURL
$curl = curl_init();
//Will return the response, if false it prints the response
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
//Set the url, in this case Tallyfy's organization tasks endpoint
curl_setopt($curl, CURLOPT_URL, 'https://api.tallyfy.com/organizations/{ My Tallyfy organization id }/tasks');
//Set the header of our Tallyfy API request to our authenticating information
curl_setopt($curl, CURLOPT_HTTPHEADER, array(
'accept: application/json',
'content-type: application/json',
'authorization: Bearer { My Tallyfy authorization token }',
'X-Tallyfy-Client: SlackBot Demo'
));
//Execute cURL
$result = curl_exec($curl);
//Close the cURL
curl_close($curl);
Step 6: Process the Tasks Returned by the Tallyfy API
Now we have to look at the tasks returned by our API request, and fashion them in a way that Slack can understand. To do this, we will convert tasks into an array of Slack attachments and add them to a body we cURL to Slack. This will basically be a JSON that looks something like this:
{
"mkdwn" : true,
"text" : "*Tasks to do:*",
"attachments":
[
{
"color" : "#3DB75C",
"text" : "This is a task!"
},{
"color" : "#3DB75C",
"text" : "This is also a task!"
}
]
}
To do this, we first have to turn our result from the Tallyfy API into an array object.
...
// Execute cURL
$result = curl_exec($curl);
//Close the cURL
curl_close($curl);
//Decode our cURL result into an array
$json = json_decode($result, true);
After we’ve turned our result into an array, we have to grab the value for the “data” key.
...
// Execute cURL
$result = curl_exec($curl);
//Close the cURL
curl_close($curl);
//Decode our cURL result into an array
$json = json_decode($result, true);
//Get the Tallyfy data from the JSON array
$data = $json['data'];
From here, we can now create an empty “attachments” array. We then loop through each task in the Tallyfy data and add it as an attachment to our attackments array.
...
// Execute cURL
$result = curl_exec($curl);
//Close the cURL
curl_close($curl);
//Decode our cURL result into an array
$json = json_decode($result, true);
//Get the Tallyfy data from the JSON array
$data = $json['data'];
$attachments = array();
foreach ($data as $task) {
$task_name = $task['title'];
array_push($attachments, array(
'color' => '#3DB75C',
'text' => $task_name
)
);
}
Now we have a bunch of attachments we want to send to slack. We can use this attachments array to create that cURL body to send to Slack we mentioned earlier.
...
// Execute cURL
$result = curl_exec($curl);
//Close the cURL
curl_close($curl);
//Decode our cURL result into an array
$json = json_decode($result, true);
//Get the Tallyfy data from the JSON array
$data = $json['data'];
$attachments = array();
foreach ($data as $task) {
$task_name = $task['title'];
array_push($attachments, array(
'color' => '#3DB75C',
'text' => $task_name
)
);
}
//Create an HTTP body to pass in our Slack POST
$slack_body = array(
//Setting mkdwn to true allows us to bold substrings by encasing them in asterisks
'mkdwn' => true,
'text' => "*Tasks to do:*",
'attachments' => $attachments
);
We are now ready to send our to-do attachments back to Slack!
Step 7: Send the Attachments Back to Slack
Now that we’ve processed the tasks returned by the Tallyfy API, we can send our to-do attachments back to Slack. Consistent with the rest of this tutorial, we will be using cURL to send the HTTP body we made back to Slack. This is simply a few lines of code we can basically copy and paste to the bottom of our php file.
...
//Now we have everything we need to post our HTTP body to slack and print a new message
//Reinitialize our cURL
$curl = curl_init();
//Set the cURL to the appropriate webhook (the one we saved earlier in Step 4: "Activate Incoming Webhooks") url for your app and set the cURL to a POST request
curl_setopt($curl, CURLOPT_URL, 'https://hooks.slack.com/services/{ Your slack webhook }');
curl_setopt($curl, CURLOPT_POST, 1);
//Set the cURL's body to a JSON encoding of our slack_body
curl_setopt($curl, CURLOPT_POSTFIELDS, json_encode($slack_body));
//Receive server response ...
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
//Execute and close our cURL
curl_exec($curl);
curl_close($curl);
}
?>
Now, your code should work fine. When you’re finished, your php file should look something like this:
<?php
//Initiate cURL
$curl = curl_init();
//Will return the response, if false it prints the response
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
//Set the url, in this case Tallyfy's organization tasks endpoint
curl_setopt($curl, CURLOPT_URL, 'https://api.tallyfy.com/organizations/{ My Tallyfy organization id }/tasks');
//Set the header of our Tallyfy API request to our authenticating information
curl_setopt($curl, CURLOPT_HTTPHEADER, array(
'accept: application/json',
'content-type: application/json',
'authorization: Bearer { My Tallyfy authorization token }',
'X-Tallyfy-Client: SlackBot Demo'
));
// Execute cURL
$result = curl_exec($curl);
//Close the cURL
curl_close($curl);
//Decode our cURL result into an array
$json = json_decode($result, true);
//Get the fact, as a string, from the JSON array
$data = $json['data'];
$attachments = array();
foreach ($data as $task) {
$task_name = $task['title'];
array_push($attachments, array(
'color' => '#3DB75C',
'text' => $task_name
)
);
}
//Create an HTTP body to be passed in our Slack POST
$slack_body = array(
//Setting mkdwn to true allows us to bold substrings by encasing them in asterisks
'mkdwn' => true,
'text' => "*Tasks to do:*",
'attachments' => $attachments
);
//Now we have everything we need to post our HTTP body to slack and print a new message
//Reinitialize our cURL
$curl = curl_init();
//Set the cURL to the appropriate webhook (the one we saved earlier in Step 4: "Activate Incoming Webhooks") url for your app and set the cURL to a POST request
curl_setopt($curl, CURLOPT_URL, 'https://hooks.slack.com/services/{ Your slack webhook }');
curl_setopt($curl, CURLOPT_POST, 1);
//Set the cURL's body to a JSON encoding of our slack_body
curl_setopt($curl, CURLOPT_POSTFIELDS, json_encode($slack_body));
// Receive server response ...
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
//Execute and close our cURL
curl_exec($curl);
curl_close($curl);
?>
Although we’re done coding, there is still one more step we have to do to make sure we can use our slash command correctly.
Step 8: Uninstall and Reinstall Slack Bot
Now, whatever order I would do the above steps in, I found that I always have to uninstall and reinstall the Slack Bot from my Slack channel. Failure to do so means that the channel will not recognize our slash command.
To uninstall and reinstall, simply go to the channel you originally installed the Slack Bot to. From here, you’ll see an automated message that looks like “added an integration to this channel: Todo Bot”. Click on the name of the bot and choose “Settings.” From this app settings page, you can now “Remove App” and then install the app to your channel again.
Note: any time you install an app to a new channel, the webhook URL will change. This includes uninstalling and reinstalling to a channel. Be sure to update your php file for the new webhook as seen in step 7!
Once you reinstall your Slack Bot, we should be able to call “/todo” and see our list of Tallyfy tasks!
Creating Other Slack Bots with this Code
Now, for this example, I used the Tallyfy API as that is the API of the company I work for. I’m familiar with it and it was easy to use. This code snippet I’ve provided could, of course, be altered to relay information from any API back to your Slack channel. To prove this point, the following code snippet is a slightly altered version of our Todo Bot code that will relay random, useless facts instead of our organization’s tasks.
<?php
//Initiate cURL
$curl = curl_init();
// Will return the response, if false it prints the response
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
// Set the url, in this case the random fact generator response as JSON
curl_setopt($curl, CURLOPT_URL, 'https://uselessfacts.jsph.pl/random.json?language=en');
// Execute cURL
$result = curl_exec($curl);
//Close the cURL
curl_close($curl);
//Decode our cURL result into an array
$json = json_decode($result, true);
//Get the fact, as a string, from the JSON array
$fact_text = $json['text'];
//Create an HTTP body to be passed in our Slack POST
$slack_body = array(
//Setting mkdwn to true allows us to bold substrings by encasing them in asterisks
'mkdwn' => true,
'text' => '*Random fact:*',
'attachments' => array(
array(
//You can change the attachment color to whatever you'd like
'color' => '#3DB75C',
'text' => $fact_text
)
)
);
//Now we have everything we need to post our HTTP body to slack and print a new message
//Reinitialize our cURL
$curl = curl_init();
//Set the cURL to the appropriate webhook (the one we saved earlier in Step 4: "Activate Incoming Webhooks") url for your app and set the cURL to a POST request
curl_setopt($curl, CURLOPT_URL, 'https://hooks.slack.com/services/{ Your slack webhook }');
curl_setopt($curl, CURLOPT_POST, 1);
//Set the cURL's body to a JSON encoding of our slack_body
curl_setopt($curl, CURLOPT_POSTFIELDS, json_encode($slack_body));
// Receive server response ...
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
//Execute and close our cURL
curl_exec($curl);
curl_close($curl);
?>
The end result looks something like this!
The Tallyfy Slack App
Although we created a Slack Bot that utilizes Tallyfy’s API in this tutorial, there is actually a much more comprehensive Slack App for Tallyfy users! If you are interested in integrating task management to your Slack channel, be sure to check out Tallyfy and their new Slack App!
More info about the Tallyfy Slack App can be found here. If you’re interested in giving Tallyfy a shot, be sure to click here.